Recommended Resources
Contents
Recommended Resources#
Table of Contents
Books and tutorials#
Learn Python Programming#
programiz.com: Learn Python Programming
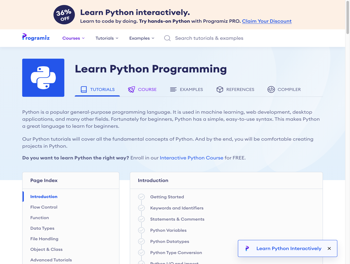
A tutorial site with an easy to navigate interface and lessons with nice looking code samples and optional videos. The site also features a reference section as well as a library of examples.
Warning
Lots of annoying banner and pop-up ads. Not recommended without an ad blocker.
Section recommendations
-
Skip Getting Started
-
Skip Modules and Package
Python Practice Book#
Section recommendations
Python for you and me#
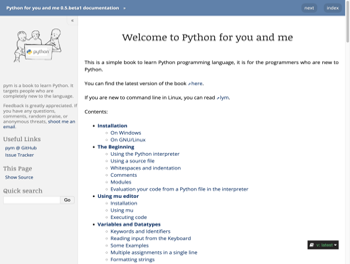
A nicely formatted online book to learn Python targeted at beginners with plenty of code samples.
A Whirlwind Tour of Python#
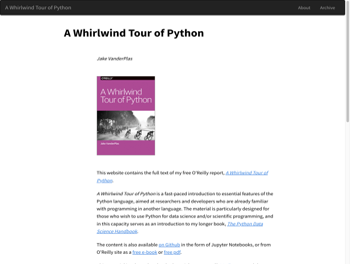
A concise and clearly written online book with nice looking code samples.
Exercises and Practice#
Codewars#
A platform that helps you learn, train, and improve your coding skills by solving programming tasks of many types and difficulty levels.