Program Flow
Contents
Program Flow#
In this lesson we are going to do an exercise seeing code the way that Python does. We’re going to do this by pretending that as Python executes each statement, it is playing a video game.
Review#
If Python was a game#
Assigning Variables#
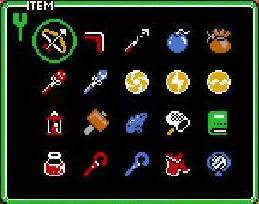
If executing Python was like playing a video game, the step of assigning variables would be like collecting inventory.
Let’s walk through the following example code.
.
1character = "Mario"
2coins = 25
3potions = 5
4objects = [
5 "iron key",
6]
Defining functions#
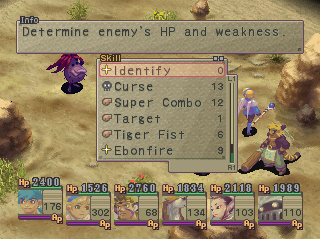
If executing Python was like playing a video game, the step of defining functions would be like acquiring skills or abilities.
Let’s walk through an example.
.
1character = "Mario"
2coins = 25
3potions = 5
4objects = [
5 "iron key",
6]
7
8def hide():
9 print("You cast a concealment spell. *poof* Nobody can see you")
10
11def pick_up(name):
12 objects.append(name)
13 print("You picked up a", name, ".")
Calling functions#
If executing Python was like playing a video game, the step of calling functions would be like using your skills or abilities.
Let’s walk through an example.
1character = "Mario"
2coins = 25
3potions = 5
4objects = [
5 "iron key",
6]
7
8def hide():
9 print("You cast a concealment spell. *poof* Nobody can see you")
10
11def pick_up(name):
12 objects.append(name)
13 print("You picked up a", name, ".")
14
15hide()
The "You cast a spell..."
message doesn’t get printed until you call
the function on line 15
.
Calling more functions#
1character = "Mario"
2coins = 25
3potions = 5
4objects = [
5 "iron key",
6]
7
8def hide():
9 print("You cast a concealment spell. *poof* Nobody can see you")
10
11def pick_up(name):
12 objects.append(name)
13 print("You picked up a", name, ".")
14
15hide()
16pick_up("silver key")
17pick_up("spellbook")
The pick_up
function changed the value of the objects
variable.
Referencing variables#
1character = "Mario"
2coins = 25
3potions = 5
4objects = [
5 "iron key",
6]
7
8def hide():
9 print("You cast a concealment spell. *poof* Nobody can see you")
10
11def pick_up(name):
12 objects.append(name)
13 print("You picked up a", name, ".")
14
15hide()
16pick_up("silver key")
17pick_up("spellbook")
18
19print(character, "has finished Level One.")
An action is preformed on a variable when it is referenced. So even though the
character
variable is assigned on line 1
, it isn’t referenced until line 19
.